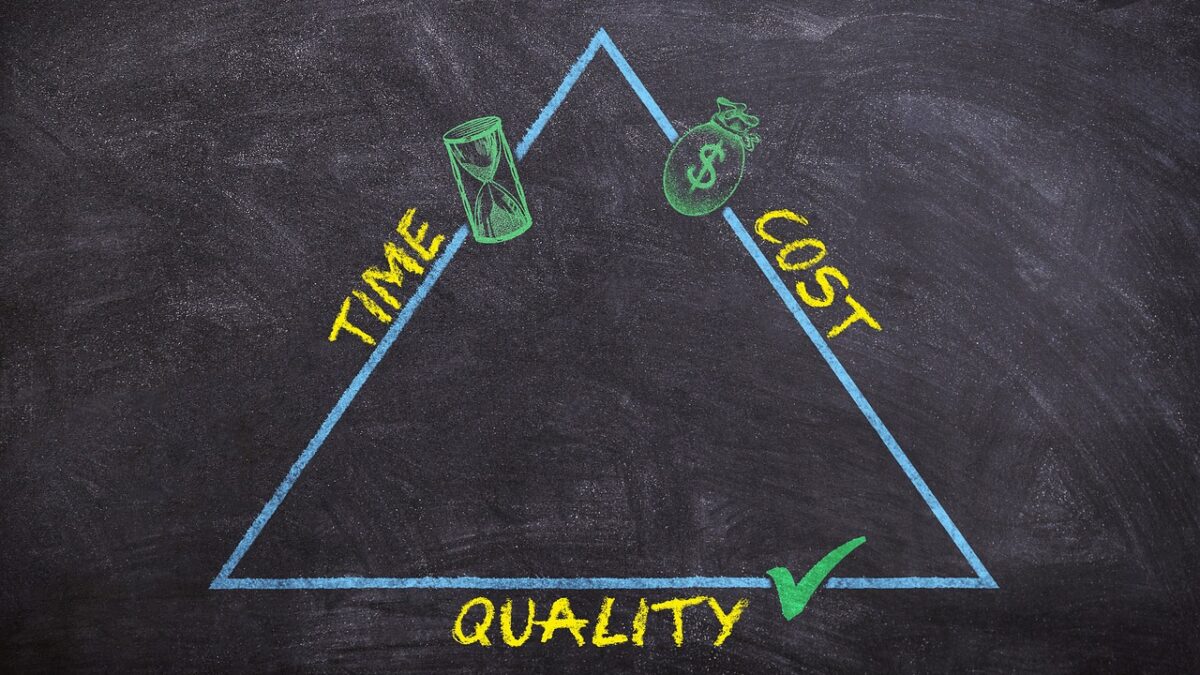
10 Best Practices for Writing Efficient and Maintainable Code
Writing efficient and maintainable code is crucial for every developer. It ensures that your code is easy to understand, debug, and modify, which ultimately saves time and effort. In this blog post, we’ll discuss 10 best practices that can help you write efficient and maintainable code.
1. **Use meaningful variable and function names**: Choose descriptive names that accurately represent the purpose of the variable or function. This makes your code easier to understand and maintain. For example, instead of using `x` or `temp`, use `num_users` or `calculate_average`.
2. **Follow a consistent coding style**: Consistency in coding style makes your code easier to read and understand. Use meaningful variable names. Use camelCase for variables and PascalCase for functions and classes.
3. **Write modular code**: Break your code into smaller, reusable functions or modules. This makes your code more maintainable and easier to test and debug.
4. **Avoid code duplication**: Duplicated code can lead to inconsistencies and makes it harder to update your code in the future. Instead, create reusable functions or modules to avoid duplication.
5. **Keep functions and classes small**: Functions and classes that are too long and complex can be difficult to understand and maintain. Aim to keep your functions and classes small and focused on a single task.
6. **Use comments to explain complex code**: If you have complex code that may be difficult to understand, use comments to explain the logic and purpose of the code. This helps other developers (and yourself) understand the code more easily.
7. **Write self-documenting code**: Aim to write code that is self-explanatory, without the need for excessive comments. Use descriptive variable and function names, and structure your code in a logical and readable way.
8. **Use version control**: Version control systems like Git help you track changes in your code and collaborate with other developers. This makes your code more maintainable and easier to update.
9. **Write unit tests**: Unit tests help ensure that your code works as expected and can catch bugs early in the development process. Writing tests also makes your code more maintainable, as it allows you to refactor your code with confidence.
10. **Regularly refactor your code**: Refactoring is the process of improving the structure and design of your code without changing its external behavior. Regularly reviewing and refactoring your code helps keep it efficient and maintainable.
Writing efficient and maintainable code is crucial for every developer. By following these 10 best practices, you can improve the quality of your code and make it easier to understand, debug, and modify.